이전 포스팅이 있으니 먼저 진행해보시기를 추천드립니다.!!
https://sas-study.tistory.com/356
오늘은 지난 컨트롤러 작성한 부분에서 Spring Security 를 적용해보겠습니다.
프로젝트 구조는 다음과 같습니다.
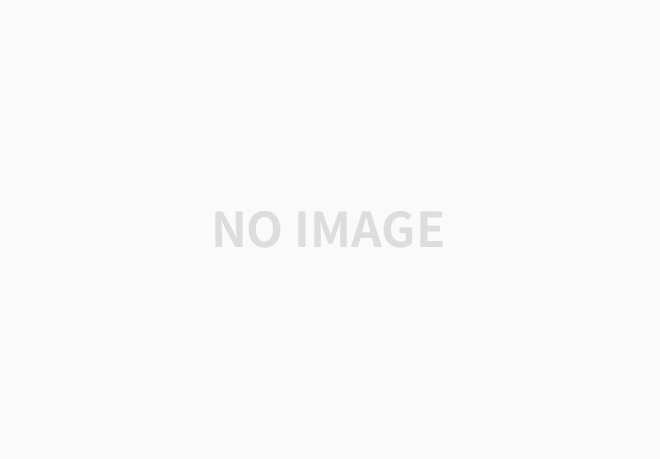
configuration 패키지에 SecurityConfig 자바파일을 추가해주었습니다.
소스 살펴보기
TestController.java
@RequiredArgsConstructor
@RequestMapping("/api/v1/test")
@RestController
public class TestController {
private final TestService testService;
@GetMapping("/permit-all")
public Object getTest() throws Exception {
return testService.getTest();
}
@GetMapping("/auth")
public Object getTest2() throws Exception {
return testService.getTest2();
}
}
이전 포스팅을 보면 다음과 같이 컨트롤러 RequestMapping을 만들어 놓았습니다.
/permit-all : 스프링 시큐리티에 관계없이 모두 접근 가능
/auth : 스프링 시큐리티 내에서 관리되어야할 URL
이것을 설정하게 도와줄 SecurityConfig.java 파일을 만듭니다.
SecurityConfig.java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.cors().and()
.csrf().disable()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.antMatchers("/api/v1/test/permit-all").permitAll()
.antMatchers("/api/v1/test/auth").authenticated()
.antMatchers("/**").authenticated()
.anyRequest().permitAll()
.and()
.formLogin().disable()
;
}
}
- @Configuration : 자바로 진행하는 설정 클래스에 붙이는 어노테이션으로 스프링 빈으로 만들고 스프링 프로젝트가 시작될 때 스프링 시큐리티 설정내용에 반영되도록 한다.
- @EnableWebSecurity : 스프링 시큐리티를 활성화하는 어노테이션이다.
- WebSecurityConfigureAdapter : 스프링 시큐리티 설정관련 클래스로 커스텀 설정클래스가 이 클래스의 메소드를 오버라이딩하여 설정하여야 스프링 시큐리티에 반영된다.
오늘 해야할 일은 /permit-all과 /auth를 스프링 시큐리티에서 구분하여 인증 절차를 거치는 작업입니다. 이것에 초점을 맞추었습니다.
허나 cors, csrf, session과 같은 정책은 설정 초기에 잡는 편이기 때문에 위와 같이 추가해두었습니다.
- cors().and() : 이 구문은 안에 들어가보면 주석으로 내용이 나와있지만 CorsFilter라는 필터가 존재하는데 이를 활성화 시키는 작업입니다.
- csrf().disable() : 세션을 사용하지 않고 JWT 토큰을 활용하여 진행하고 REST API를 만드는 작업이기때문에 csrf 사용은 disable 처리합니다.
- sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS) : 현재 스프링 시큐리티에서 세션을 관리하지 않겠다는 뜻입니다. 서버에서 관리되는 세션 없이 클라이언트에서 요청하는 헤더에 token을 담아보낸다면 서버에서 토큰을 확인하여 인증하는 방식을 사용할 것이므로 서버에서 관리되어야할 세션이 필요없어지게 됩니다.
- authorizeRequests() : 이제부터 인증절차에 대한 설정을 진행하겠다는 것입니다.
- antMatchers() : 특정 URL 에 대해서 어떻게 인증처리를 할지 결정합니다.
- permitAll() : 스프링 시큐리티에서 인증이 되지 않더라도 통과시켜 누구에게나 사용을 열어줍니다.
- authenticated() : 요청내에 스프링 시큐리티 컨텍스트 내에서 인증이 완료되어야 api를 사용할 수 있습니다. 인증이 되지 않은 요청은 403(Forbidden)이 내려집니다.
그리하여 /api/v1/test/permit-all 이라는 url로 들어오는 모든 요청은 200, /api/v1/test/auth 라는 url로 들어오는 요청을 인증을 거친 후, 200/403의 결과가 나올 것입니다.
또한가지
.antMatchers("/api/v1/test/auth").anonymous() |
이런식으로 .anonymous로 설정할 수 있는데, 주의할 점이 이렇게 설정되어있다면 유저의 상태가 anonymous일 때만 호출이 가능합니다. 즉, 인증된 유저는 접근할 수 없는 요청이 되어버리니 주의하시기 바랍니다.
이렇게 설정을 하고 난 후, 서버를 실행하여 확인해보면!!
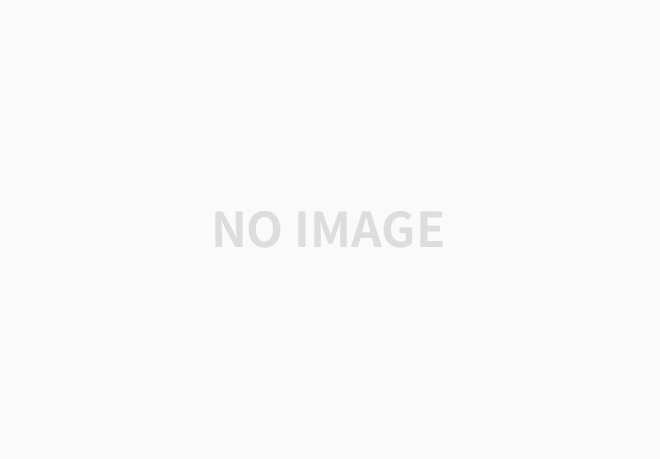
다음과 같이 /auth는 아직 인증로직이 개발되지 못했으니 무조건 403(Forbidden)이 떨어질 것이고, /permit-all은 무조건 통과되니 200(ok)가 떨어질 것입니다.
다음 포스팅에서는 인증로직을 거처서 /auth가 통과되도록 설정해보겠습니다.
감사합니다.